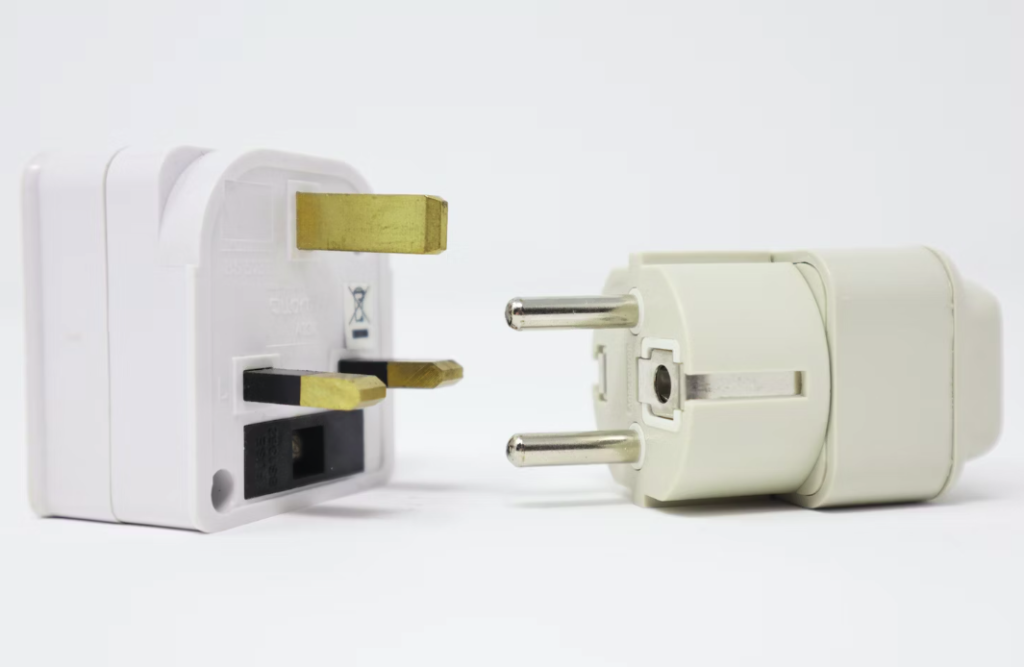
Introduction
In the ever-evolving world of software, one constant is the need to integrate or work with legacy systems. Often, you’ll come across situations where you need to interact with old codebases, third-party libraries, or systems you cannot modify. This is where design patterns, specifically the Adapter pattern, come to the rescue.
The Adapter pattern is a structural design pattern that allows two incompatible interfaces to work together. Instead of rewriting code or abandoning legacy systems, this pattern provides a middle ground, bridging the old with the new. Today, we’ll walk through an example illustrating the benefits of this pattern.
The Problem
Imagine you’re building a system for a library. The existing infrastructure provides book information in XML format, but your new system operates on JSON. The legacy system is unmodifiable, so how can we bridge this data format gap?
Take a look at the method in the legacy system:
func (l LegacyLibService) GetBook() XmlString {
return `
<book>
<title>The Great Gatsby</title>
<author>F. Scott Fitzgerald</author>
<year>1925</year>
</book>
`
}
Our task is to seamlessly fetch this book data in JSON format for our modern system.
The Adapter Pattern to the Rescue
Enter the LegacyLibAdapter
. This structure adapts the XML output from the legacy system to a JSON format that the modern system expects.
Let’s see this in action:
type LegacyLibAdapter struct {
adaptee *LegacyLibService
}
func (l LegacyLibAdapter) GetBook() (JsonString, error) {
var xmlBook Book
xmlResponse := l.adaptee.GetBook()
if err := xml.Unmarshal([]byte(xmlResponse), &xmlBook); err != nil {
// Handle error
return "", err
}
jsonResponse, err := json.Marshal(xmlBook)
if err != nil {
// Handle error
return "", err
}
return JsonString(jsonResponse), nil
}
The beauty of this solution is in its simplicity. The Adapter pattern helps retain the functionality of the legacy system while making it compatible with the new system.
Why is the Adapter Pattern Crucial?
- Protects Against Redundant Work: Instead of rewriting a potentially vast codebase, you’re simply creating a bridge. This not only saves time but also avoids introducing new bugs into a working system.
- Promotes Decoupling: The modern system remains decoupled from the legacy system, relying only on the
LibraryService
interface. This separation ensures flexibility and promotes the Open/Closed principle of software design, wherein software entities should be open for extension but closed for modification. - Enhances Interoperability: It’s common to interface with third-party libraries or APIs that you cannot change. The Adapter pattern ensures you can always make them work in your system.
In our example:
var lib LibraryService = NewLibraryService()
jsonRes, err := lib.GetBook()
// Output: Json book output: {"Title":"The Great Gatsby","Author":"F. Scott Fitzgerald","Year":"1925"}
The application code remains agnostic of the underlying XML to JSON conversion, showcasing the pattern’s elegance.
Closing Thoughts
The Adapter pattern is a testament to the resilience and adaptability required in software engineering. Instead of avoiding or discarding the old, it shows us a way to embrace it and bring it along into the new age. Whether you’re dealing with legacy code, third-party libraries, or systems you can’t change, remember the Adapter pattern, and you’ll always find a way to bridge the gap.
Happy Coding!