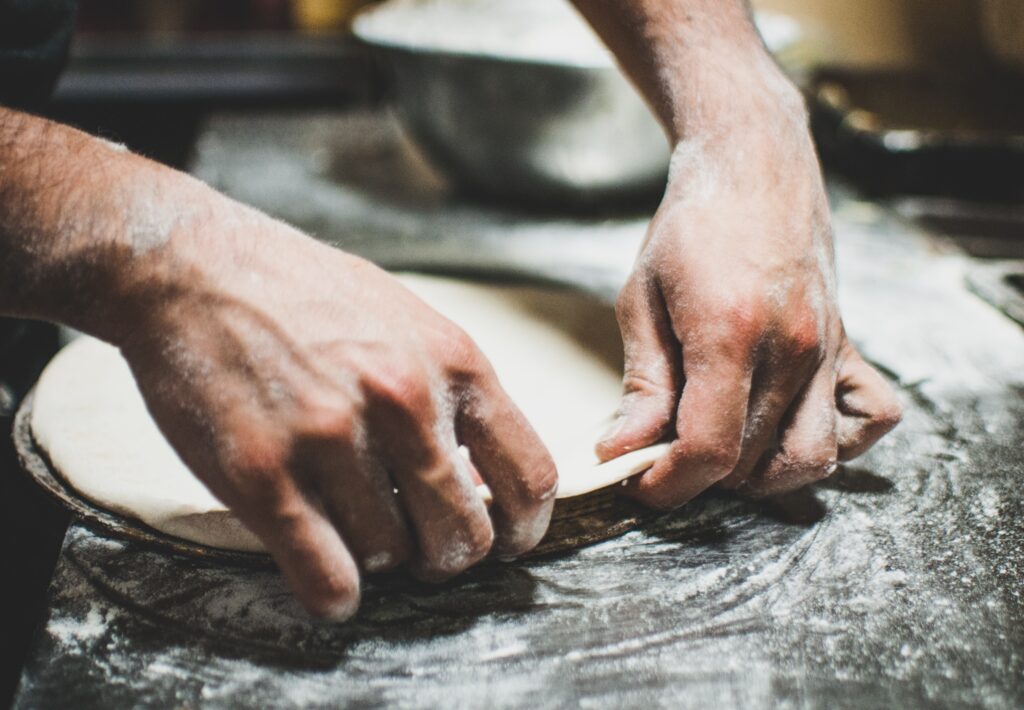
Introduction
In the software design world, the Builder pattern stands out for its unique ability to encapsulate the construction process of complex objects. But, why just read about it when we can delve into it with a delicious analogy? Let’s explore the Builder pattern with an example everyone loves – pizza!
The Scenario:
Imagine you’re at a pizza parlor where they let you design your own pizza. You choose the crust, the sauce, the size, and heap on your favorite toppings. Just like crafting the perfect pie, creating complex objects in programming can benefit from a structured approach. This is where our Builder pattern shines!
Diving into the Code:
To start, let’s define our pizza attributes: PizzaBase
, PizzaSauce
, PizzaSize
, and PizzaTopping
. These represent all the possible components of our pizza.
ThickCrust PizzaBase = "Thick Crust"
StuffedCrust PizzaBase = "Stuffed Crust"
)
// ... Similarly for sauce, size, and toppings.
Now, just like how a pizza is more than a sum of its parts, our Pizza
struct encompasses all these components:
type Pizza struct {
base PizzaBase
sauce PizzaSauce
size PizzaSize
toppings []PizzaTopping
}
So, where does the Builder pattern come into play? If you’ve ever been to a custom pizza shop, you’ll know you don’t just list out all your preferences at once. You go step-by-step. The Builder
struct encapsulates this step-by-step creation process.
type Builder struct {
pizza Pizza
}
The builder has methods to set each component, and every method returns the builder itself. This enables method chaining – a fluent way of setting properties.
func (b *Builder) Base(base PizzaBase) *Builder {
b.pizza.base = base
return b
}
// ... Similarly for sauce, toppings, and size.
Once you’ve chosen all your pizza’s components, it’s time to bake it! The GetPizza()
method does this, ensuring all mandatory components are in place before serving up your pizza.
func (b *Builder) GetPizza() (*Pizza, error) {
// Checks for pizza completeness and then returns the pizza.
if b.pizza.base == "" || b.pizza.sauce == "" || b.pizza.size == "" {
return nil, errors.New(fmt.Sprintf("Called 'GetPizza()' on unfinished pizza: %v", b.pizza))
}
return &b.pizza, nil
}
Finally, the main event:
func main() {
var b Builder
b.NewBuilder().Base(ThinCrust).Topping(Pepperoni).Topping(Mushrooms).Topping(Olives).Sauce(Tomato).Size(Large)
pizza, err := b.GetPizza()
if err != nil {
fmt.Printf("Error while getting pizza: %v\\n", err)
os.Exit(1)
}
fmt.Printf("Your Pizza is ready: %v\\n", pizza)
}
Wrapping Up:
Just like how you enjoy crafting your perfect pizza with layers of flavors, the Builder pattern allows for a structured and methodical approach to object construction. It separates the creation from representation, ensuring both clarity and flexibility. So, the next time you bite into a custom pizza, maybe you’ll think of the Builder pattern – a delightful blend of design and functionality!
Happy coding and bon appétit!
Email: [email protected]
Linkedin: https://www.linkedin.com/in/eric-howard-8a4166127/